Introduction
In this post, I’ll share how I resolved a common issue on Vercel where refreshing a non-source page results in a “Page Not Found” error. This was particularly problematic on my blog at www.walterclayton.com.
Static Website Solution
Problem:
When refreshing a page that is not the home URL (e.g., https://www.walterclayton.com/about), I encountered a “Page Not Found” error. The page rendered perfectly when navigating through the navbar but failed upon direct refresh.
Solution:
To fix this, you need to add a vercel.json file to the root directory of your project with the following content:
{
"rewrites": [
{
"source": "/(.*)",
"destination": "/index.html"
}
]
}
This configuration ensures all requests are routed through your index page, allowing your client-side router to handle the navigation.
Web App Solution
This issue took me a week to resolve as I was initially running a full-stack project on Vercel. Here’s how I addressed it.
Problem:
For a full-stack project with both frontend and backend (e.g., using Node, Express, MongoDB, React, and Vite), I faced the same issue. However, running both servers as separate projects on Vercel and adding the vercel.json file only to the client project’s root directory proved to be the solution.
Solution:
Add a Catch-All Route Handler in Express:
// The "catchall" handler: for any request that doesn't
// match one above, send back React's index.html file.
app.get("*", (req, res) => {
res.sendFile(path.join(__dirname, "../client/dist", "index.html"));
});
Add vercel.json for Rewrites in the Client Root Directory:
{
"rewrites": [
{
"source": "/(.*)",
"destination": "/index.html"
}
]
}
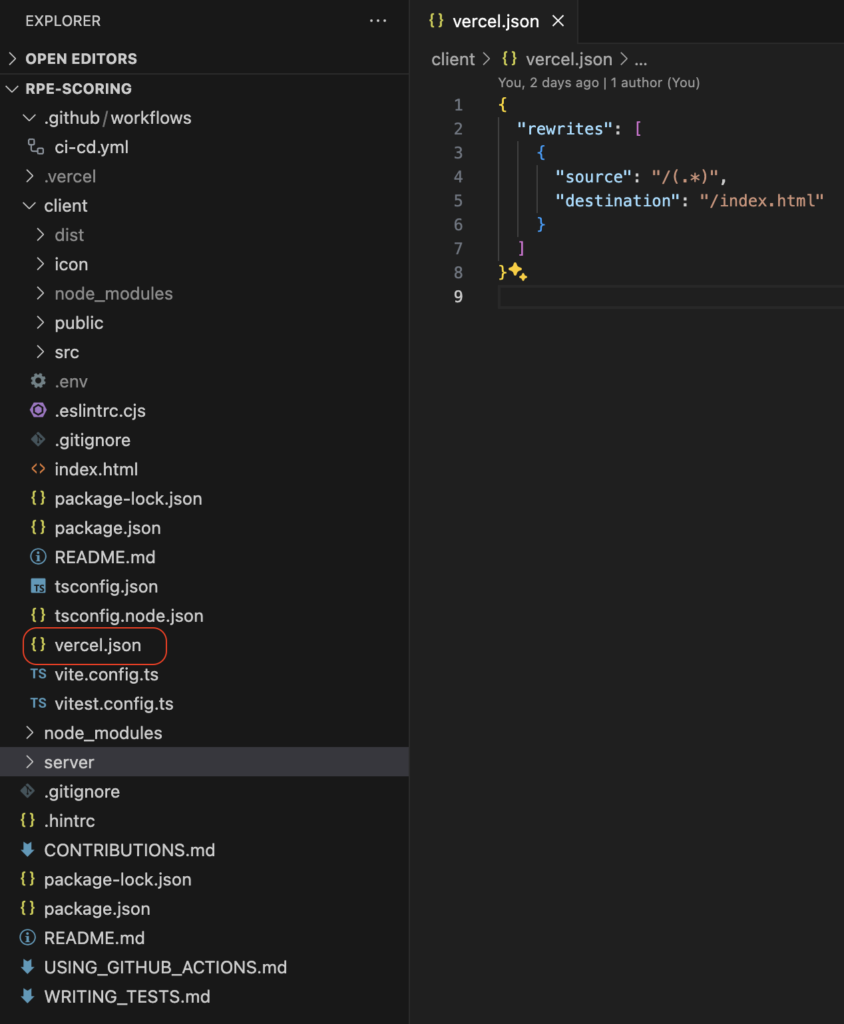
Initially, I encountered an error from the client side (npm run client exited with code 0). This led me to reconsider and set up the project where both front and back ends run concurrently using the concurrently package. Eventually, the realization struck that the vercel.json file should indeed be in the root directory of the client project, similar to the static website solution.
Conclusion:
By following these steps, you can ensure that your Vercel-hosted applications handle client-side routes correctly, even when pages are refreshed directly. This solution applies to both static websites and full-stack applications.
Hope this helps any of you readers out there 🤙
Leave a Reply